Logic Synthesis with VHDL
Combinational Logic
Bob Reese
Electrical Engineering Department
Mississippi State University
Converted to HTML by
MANJUNATH R. MITTHA,
MS Student, Dept. of Electrical Eng.,
Mississippi State University
Index
Logic Synthesis
Tutorial Caveats
VHDL Synthesis Subset
General Comments about VHDL Syntax
Combinational Logic Examples
Model Template
2 to 1 Mux - Using when else..
Standard Logic 1164
2 to 1 Mux Entity Declaration
2 to 1 Mux Architecture Declaration
2 to 1 Mux Architecture using Booleans
2 to 1 Mux Architecture using a Process
8 Level Priority Encoder
3 to 8 Decoder Example
A Common Error
Alternative 3 to 8 Decoder
Generic Decoder
Synthesis Boundary Conditions
Ripple Carry Adder
Ripple Carry Adder Comments
Summary
- Use of Logic Synthesis has become common industrial practice. The advantages are many:
- Technology portability
- Design Documentation
- Constraint Driven Synthesis
- Two major languages are Verilog and VHDL. This tutorial will convert logic synthesis via VHDL.
- We will split the tutorials into three parts:
- Introduction to VHDL via combinational synthesis examples
- Sequential synthesis examples (registers, finite state machines)
- System examples (combined datapath and control)
BACK TO INDEX
- Tutorial examples have been made as simple and portable as possible.
- Will stay away from topics such as parameterization which may involve vendor-dependent
features.
- Will also stay away from coding styles which involve type conversion as this tends to add
extra complications.
- Examples have been tested with the Synopsys and Viewlogic synthesis tools; most of the
synthesized schematics shown in the slides are from the Viewlogic synthesis tool. Some of
the more complex examples are only compatible with the Synopsys environment.
- In these tutorials, the suggested styles for writing synthesizable VHDL models come from
my own experience in teaching an ASIC design course for Senior/Graduate EE students.
- Coverage of VHDL packages will be light; the block structural statements and VHDL
configurations are skipped. Generics are not mentioned until late in the tutorial
since support from a synthesis point of view is vendor dependent.
- This tutorial is no substitute for a good, detailed VHDL textbook or the language reference
manual. Get one or both!!!
BACK TO INDEX
- The VHDL language has a reputation for being very complex -- that reputation is well deserved!
- Fortunately, the subset of VHDL which can be used for synthesis is SMALL -- very easy to learn.
- Primary VHDL constructs we will use for synthesis:
- signal assignment
nextstate <= HIGHWAY_GREEN
- comparisons
= (equal), /= (not equal),
> (greater than), < (less than)
<= ( less than or equal), >= (greater than or equal)
- logical operators
(and, xor, or, nand, nor, xnor, not )
- 'if' statement
if ( presentstate = CHECK_CAR ) then ....
end if | elsif ....
- 'for' statement (used for looping in creating arrays of elements)
- Other constructs are 'when else', 'case' , 'wait '. Also ":=" for
variable assignment.
BACK TO INDEX
- Most syntax details will be introduced on an 'as-needed' basis.
- The full syntax of a statement type including all of its various options will often NOT be
presented; instead, these will be introduced via examples as the tutorial progresses.
- There are many language details which will be glossed over or simply skipped for
the sake of brevity.
- Generalities:
- VHDL is not case sensitive.
- The semicolon is used to indicate termination of a statement.
- Two dashes ('--') are used to indicate the start of a comment.
- Identifiers must begin with a letter, subsequent characters must be alphanumeric
or '_' (underscore).
- VHDL is a strongly typed language. There is very little automatic type conversion; most
operations have to operate on common types. Operator overloading is supported in
which a function or procedure can be defined differently for different argument lists.
BACK TO INDEX
- We will go through some combinational examples to introduce you to the synthesizable subset
of VHDL. Usually, we will demonstrate multiple methods of implementing the same design.
- Examples are:
- 2 to 1 Mux
- 8*level priority circuit
- 3 to 8 Decoder
- Synthesis boundary conditions
- Ripple-carry adder
BACK TO INDEX
entity model_name is
port
(
list of inputs and outputs
);
end model_name;
architecture architecture_name of model_name is
begin
...
VHDL concurrent statements
....
end architecture_name ;
BACK TO INDEX
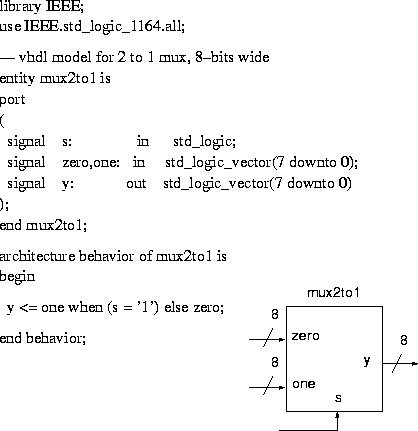
BACK TO INDEX
library IEEE;
use IEEE.std_logic_1164.all;
- The LIBRARY statement is used to reference a group of previously defined VHDL design units
(other entities or groups procedures/functions known as 'packages'.
- The USE statement specifies what entities or packages to use out of this library; in this case
'USE IEEE.std_logic_1164.all' imports all procedures/functions in the std_logic_1164 package.
- The std_logic_1164 package defines a multi-valued logic system which will be used as
the data types for the signals defined in our examples.
- The VHDL language definition had a built-in bit type which only supported two values,
'1' and '0' which was insufficient for modeling and synthesis applications.
- The 1164 standard defines a 9-valued logic system; only 4 of these have meaning
for synthesis:
'1', '0', 'Z' ( high impedance), '-' (don't care).
- The 1164 single bit type std_logic and vector type std_logic_vector (for busses) will be used
for all signal types in the tutorial examples.
BACK TO INDEX
entity mux2to1 is
port
(
signal s: in std_logic;
signal zero,one: in std_logic_vector(7 downto 0);
signal y: out std_logic_vector(7 downto 0)
);
end mux2to1;
- The entity declaration defines the external interface for the model.
- The port list defines the external signals. The signal definition consists of the signal name,
mode, and type.
- For synthesis purposes (and for this tutorial), the mode can be either in, out or inout.
- In this tutorial, the signal types will be either std_logic (single bit) or std_logic_vector (busses).
- The array specification on the std_logic_vector type defines the width of signal:
std_logic_vector (7 downto 0) (descending range)
std_logic_vector (0 to 7) (ascending range)
Both of these are 8-bit wide signals. The descending/ascending range declaration will
affect assignment statements such as:
y <= "11110000";
For descending range, y(7) is '1'; for ascending range y(0) is '1'.
BACK TO INDEX
architecture behavior of mux2to1 is
begin
y <= one when (s = '1') else zero;
end behavior;
- The architecture block specifies the model functionality.
- The architecture name is user-defined. Multiple architectures can be defined for the
same entity. VHDL configurations can be used to specify which architecture to use for
a particular entity.
- This tutorial will only use one architecture per entity and it will always be called behavior .
- The 'when ... else' statement is a conditional signal assignment statement.
'When ... else' statements can be chained such as:
......................signal_name <= value1 when condition1 else
........................value2 when condition2 else,
........................value N when conditionN else default_value;
- The 'when ... else' statement is a particular type of statement known as a concurrent statement as opposed to a sequential statement. The differences between concurrent and sequential statements will be discussed in more detail later.
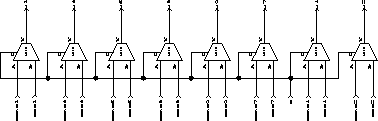
BACK TO INDEX
architecture behavior of mux2to1 is
signal temp: std_logic_vector(7 downto 0);
begin
temp <= (s, s, s, s, others => s);
y <= (temp and one) or (not temp and zero);
end behavior;
- Boolean operators are used in an assignment statement to generate the mux operation.
- The s signal cannot be used in a boolean operation with the one or zero signals because
of type mismatch (s is a std_logic type, one/zero are std_logic_vector types)
- An internal signal of type std_logic_vector called temp is declared. Note that
there is no mode declaration for internal signals. The temp signal will be used in
the boolean operation against the zero/one signals.
- Every bit of temp is to be set equal to the s signal value. An array assignment will be used;
this can take several forms:
temp <= (others => s); 'others' keyword gives default value
temp <= (s, s, s, s, s, s, s, s) ; positional assignment, 7 downto 0
temp <= (4=>s, 7=>s, 2=>s, 5=>s, 3=>s, 1=>s, 6=>s, 0=>s) ;
named assignment or combinations of the above.
BACK TO INDEX
architecture behavior of mux2to1_8 is
begin
comb: process (s, zero, one)
begin
y <= zero;
if (s = '1') then
y <= one;
end if;
end process comb;
end behavior;
- This architecture uses a process block to describe the mux operation.
- The process block itself is considered a single concurrent statement.
- Only sequential VHDL statements are allowed within a process block.
- Signal assignments are assumed to occur sequentially so that an assignment
can supercede a previous assignment to the same signal.
- 'if ... else', 'case', 'for ... loop' are sequential statements.
- The list of signals after the process block is called the sensitivity list; an event on any of
these signals will cause the process block to be evaluated during model simulation.
BACK TO INDEX
- In a process, the ordering of sequential statements which affect a common output define
the priority of those assignments.
- By using normal 'if' statements and reversing the order of the assignments we achieve
the same results as with the chained 'elsif' statements.
BACK TO INDEX
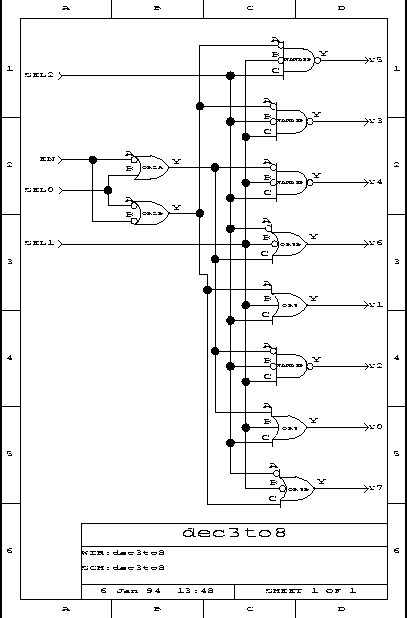
BACK TO INDEX
- When using processes, a common error is to forget to assign an output a default value.
ALL outputs should have DEFAULT values!!!!
- If there is a logical path in the model such that an output is not assigned any value
then the synthesizer will assume that the output must retain its current value and
a latch will be generated.
- Example: In dec3to8.vhd do not assign 'y' the default value of B"11111111". If en is 0,
then 'y' will not be assigned a value!
- In the new synthesized logic, all 'y' outputs are latched!
BACK TO INDEX
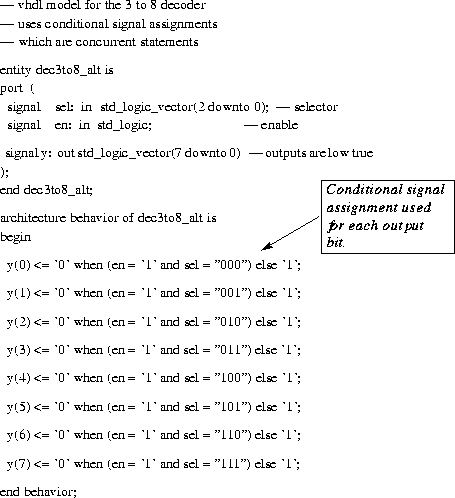
BACK TO INDEX
- Shown below is an architecture block for a generic decoder:
architecture behavior of generic_decoder is
begin
process (sel, en)
begin
y <= (others => '1') ;
for i in y'range loop
if ( en = '1' and bvtoi(To_Bitvector(sel)) = i ) then
y(i) <= '0' ;
end if ;
end loop;
end process;
end behavior;
- This architecture block can be used for any binary decoder ( 2 to 4, 3 to 8, 4 to 16, etc).
- The 'for ... loop' construct is used to repeat a sequence of statements.
- The y'range is the range of values for loop variable 'i'. The 'range attribute of
the signal 'y' is defined as the array range of the signal. In this case, 'i' will vary
from 7 to 0 if the array range of 'y' was defined as "7 downto 0".
- Other attributes useful for synthesis are: 'LEFT, 'RIGHT (left, right array indices);
'HIGH, 'LOW (max, min array indices); 'EVENT (boolean which is true if
event occurred on signal).
....
for i in y'range loop
if ( en = '1' and bvtoi(To_Bitvector(sel)) = i ) then
y(i) <= '0' ;
end if ;
- In order to compare loop variable i with the value of sel, a type conversion must be done
on sel to convert from std_logic_vector to integer.
- The Standard Logic 1164 package defines a conversion from std_logic_vector to
bit_vector (bit_vector is a primitive VHDL type).
- Unfortunately, the VHDL language standard does not define type conversions between
bit_vector and integer; these conversion functions are vendor dependent.
- 'bvtoi' is the Synopsys bit_vector to integer conversion function; 'vlb2int' is the
Viewlogic equivalent; the Cypress WARP equivalent is 'bv2i'.
BACK TO INDEX
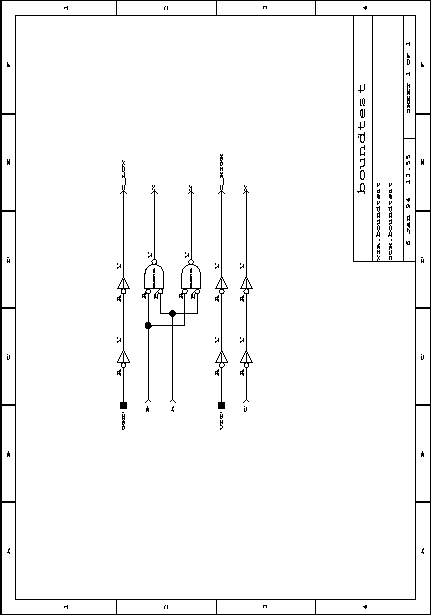
BACK TO INDEX
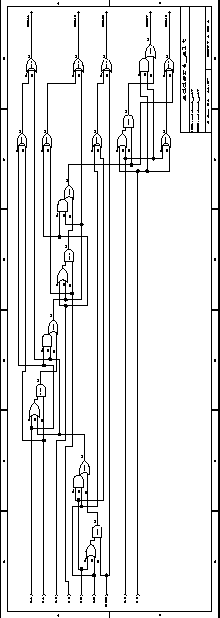
BACK TO INDEX
- The Standard Logic 1164 package does not define arithmetic operators for the std_logic type.
- Most vendors supply some sort of arithmetic package for 1164 data types.
- Some vendors also support synthesis using the '+' operation between two std_logic
signal types (Synopsis). Others provide an explicit function call (Viewlogic).
- For code portability, it is best to avoid use of vendor-specific arithmetic functions.
BACK TO INDEX
- Logic synthesis offers the following advantages:
- Faster design time, easier to modify
- The synthesis code documents the design in a more readable manner than schematics.
- Different optimization choices (area or speed)
- Several combinational VHDL examples were examined.
- Both concurrent and sequential statements can be used to specify combination logic
- which you use is up to individual preference.
BACK TO INDEX